Why do I find Ruby sexy?
I am a full time .NET developer, but I have been interested in Ruby for some time. Not very professional, but I liked this technology very much. I would like to share why I think so.
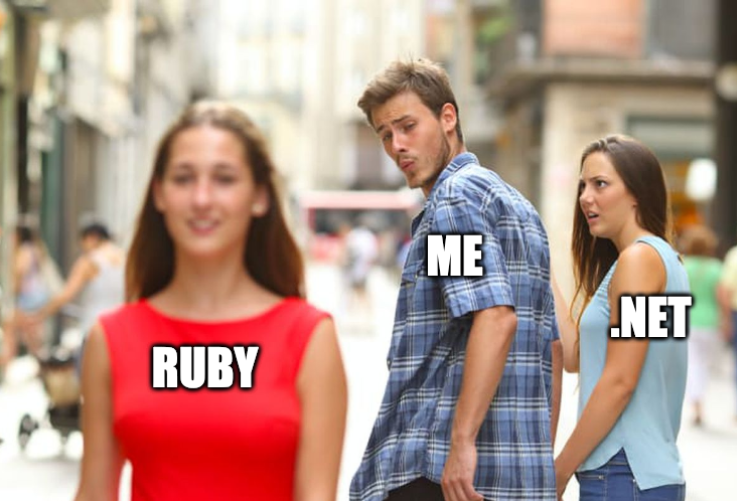
Human friendly syntax
Ruby’s creator, Yukihiro Matsumoto, said in one of the interviews: “Ruby is designed for humans, not machines”. I think that he managed to achieve his goal.
Ruby has very clean and simple syntax which makes it human friendly. I like when code is easy to read and understand.
def say_hello name
"Hello #{name}!"
end
["Mikołaj", "Tom", "Jerry"]
.map {|x| say_hello x}
.each{|x| puts x}
At first, it may look a bit like Python syntax, but unlike it, Ruby doesn’t force indentation. I believe that this is a great advantage over Python.
I’ve heard that code written in Ruby is so readable that programmers use it to discuss business logic with non-technical people. Pretty cool!
Rich standard library
The Ruby Standard Library is so rich that I can’t cover all the cool features in this post. It’s great how many useful tools we get out of the box.
Let me show you some date and time examples.
Time.now # produces current date and time
Time.now.utc # produces current date and time converted to UTC
Time.new("2021-06-01") # produces new Time object which stores given date
Time.at(1617443220) # same as above but using timestamp
Time.now.monday? # indicates if date is monday
A few more examples:
123.digits # produces [3, 2, 1]
"Test".chars # produces ["T", "e", "s", "t"]
[1, 2, 3].combination(2) # need combinations of array elements?
[1, 2, 3].permutation # or maybe permutations?
[1, nil, 2, nil, 3].compact # returns new array without nil values
[[1, 2], [3, 4]].flatten # produces [1, 2, 3, 4]
[1, 2, 3].rotate # produces [2, 3, 1]
Super handy and readable! Especially compared to e.g. the standard JavaScript library which is very poor.
I really encourage you to review the documentation because Ruby has a lot more interesting features.
Deeply ingrained testing culture
Should I write tests or not? This is obvious to Ruby programmers. They just write tests. It’s part of their culture. I am writing about it because it is not so obvious in other technologies.
Have you ever worked on any legacy projects without a single unit test? I used to and it was a traumatic experience. Ruby developers starting to work with legacy code can expect their code was somehow tested. It is better to have slow or low quality tests than none.
I’ve seen it somewhere before…
As I said before, I am a full time .NET developer. I am really accustomed to C# and its features such as Linq.
I love the way I can chain Linq methods. And guess what… Ruby has very similar feature! Take a look at this two code snippets (first is C#, second is Ruby):
new string[] { "Tom", "Jerry", "Spike" }
.Where(x => x.Contains('e'))
.Select(x => x.Length)
.Sum();
["Tom", "Jerry", "Spike"]
.filter{|x| x.include? "e"}
.map{|x| x.length}
.sum
At first glance, you can see that both pieces of code do exactly the same thing. For me both method chains are clearly traceable, readable and very elegant.
Remote work
Finally, remote work is very popular in the Ruby world. Most of the Ruby programmers worked this way before it became so popular in the pandemic era. For me personally, remote work is the best way to work. This is a huge time saver.
Final thoughts
I have to admit, Ruby programming is very enjoyable. It definitely deserves to dive deeper into its features. It’s hard for me to find something that I don’t like.
I think the next step is to look at the Ruby on Rails framework. I’ve heard a lot of good feedback about it. Especially about how efficient and productive it is to create an application using it.
If you have any interesting thoughts on this topic, let me know!